Managing foreign keys in SQL Server is a critical aspect of database design and maintenance. This article provides a comprehensive guide, covering everything from the creation of foreign keys to effective troubleshooting of any potential issues that may arise during the process. Skillfully handling foreign keys is fundamental to ensuring data integrity and maintaining a well-structured database environment. Throughout this article, we will use dbForge Studio for SQL Server as our testing ground for all the examples, demonstrating practical implementations of each concept.
Contents
- Understanding foreign keys
- Creating a foreign key
- Working with foreign keys
- Troubleshooting foreign key issues
- Checking foreign key relationships
- Conclusion
Understanding foreign keys
Foreign keys serve as a crucial mechanism when it comes to establishing and maintaining relationships between tables in relational databases. Its primary purpose is to enforce referential integrity, ensuring that data in the referenced table corresponds to existing data in the parent one. In essence, a foreign key acts as a bridge, connecting related records across different tables within a database.
A foreign key constraint (also called a referential integrity constraint) designates a column as the foreign key and establishes a relationship between that foreign key and a specified primary or unique key, called the referenced key.
Note:
- Both the foreign and the referenced keys can be located in the same table or view.
- Multiple foreign keys can be defined in a single table or view.
- A single column can be part of more than just one foreign key.
In SQL, the syntax for defining a foreign key involves specifying the field or fields to be linked and referencing the corresponding primary key in the parent table. It is a crucial component of Data Definition Language (DDL) used for table creation and modification. This relationship establishes a hierarchy between tables, designating one as the parent (containing the primary key) and the other as the child (containing the foreign key). This hierarchy forms the basis for executing operations like updates and deletions in a controlled and structured manner, preserving the integrity of the database.
Creating a foreign key
Now, we are going to venture forth to the practical aspect of handling foreign keys in the wild. dbForge Studio for SQL Server will help us tame this beast. The first task on today’s agenda is to create a foreign key.
While creating a new table in your database, you can also create the necessary foreign keys along the way:
1. In Database Explorer, connect to the instance of the database engine that contains the database to be modified.
2. Expand the connection node and then expand the database that will contain the new table.
3. Right-click the Tables node of your database and then click New Table. The Table Editor opens.
4. In the Name text box, type the table name. Optionally, add a description.
By default, the table is created in the dbo schema. To specify a different schema for the table, select the appropriate schema from the Schema drop-down list.
5. Type column names, select data types, and choose whether to allow nulls for each column, as shown in the following illustration. Optionally, add a description.
6. To specify a column as a primary key, select the check box next to the column name.
7. Switch to the Constraints tab. Then, right-click the empty space within the Constraints section and select Add foreign key.
8. In the Name field, enter the name of the constraint. Optionally, add a description and select delete and update rules if needed.
9. Select Constraint Columns as well as the corresponding Referenced Schema, Table, and Columns. In addition, you can select whether you would like the foreign key to be Enabled, Not for Replication, or to Check Existing Data.
9. Click Apply Changes.
Note: For you to be able to create a foreign key, the referenced table should have a unique index. Otherwise, dbForge Studio will prompt you to create it. Click Yes in the dialog that appears, and the unique index will be added.
Working with foreign keys
Our next step in today’s journey will be addressing the existing foreign keys, determining whether to add new ones, remove a few, adjust data in tables with foreign keys, etc.
Add foreign key in SQL Server
1. Expand the table you need, right-click the Keys folder, and choose New Foreign Key from the menu.
2. Add the required columns, select the referenced table and referenced constraint, and click Apply Changes.
You can also switch to the Constraints tab and create the constraint using the shortcut menu.
Drop foreign key in SQL Server
In order to drop a key, navigate to the constraint in Database Explorer and select Delete from the shortcut menu.
Alternatively, open the table that owns the key, switch to the Constraints tab, and choose Remove Constraint from its shortcut menu.
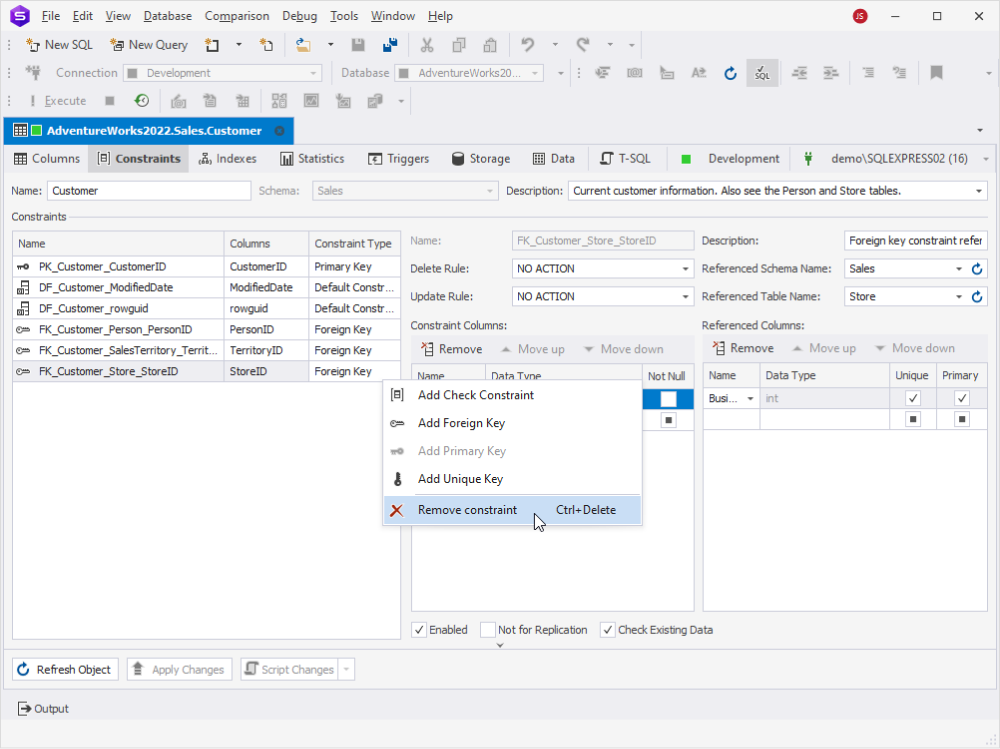
How to insert data into tables with foreign keys
Working with data in tables that have foreign keys involves a few steps to ensure data integrity. Before making any changes, it is important to understand the relationships between the tables. Specifically, you should know which tables have foreign key constraints that are being referenced by other tables, as well as which tables are acting as references to other tables.
Inserting data to a table with foreign keys does not require any particular changes in the INSERT syntax:
INSERT INTO table_name (column1, column2, ...)
VALUES (value1, value2, ...);
However, there are a couple of tips and tricks to keep in mind:
- If you are dealing with multiple tables with foreign keys, start by inserting data into the referenced table(s) first. This ensures that the data you are referencing does, in fact, exist.
- Having inserted the necessary data in the referenced table(s), you can proceed to insert data in the parent one(s).
- Make sure that the data you are inserting complies with any foreign key constraints. The value you are inserting in the parent table must exist in the referenced table.
- If the data you are inserting violates any foreign key constraints, your actions will result in an error. In this case, you will need to address this by either providing valid data or modifying the existing data in the referenced table.
- After inserting data, it is good practice to double-check that the foreign key relationships are still intact. You can do this by running appropriate SELECT queries or by using tools provided by your database management system.
How to update data in tables with foreign keys
Keeping in mind the tips above, you can update the tables with foreign keys using regular UPDATE syntax:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
How to delete data from tables with foreign keys
When deleting data from tables with foreign keys, it is important to follow the same principles as when inserting or updating data. This means considering the relationships between tables and ensuring that any foreign key constraints are taken into account.
Note: As mentioned earlier in this article, dbForge Studio for SQL Server allows you to select delete and update rules, which can automatically propagate changes to related tables. The options are:
- NO ACTION
- CASCADE
- SET NULL
- SET DEFAULT
Troubleshooting foreign key issues
Even with careful database management, encountering errors, including those involving foreign keys, is inevitable. Now, we are going to address some of the most prevalent errors associated with foreign keys and outline effective solutions, along with highlighting best practices for optimal database management.
Description | Resolution |
Foreign key mismatch | When creating a foreign key, make sure that the data types of the parent and referenced columns match (for example, both should be INT). |
Dangling foreign keys | A dangling foreign key links to a nonexistent table or column, which can lead to data integrity issues. Avoid this by creating referenced tables or columns before creating foreign key constraints. |
Missing foreign key indexes | Always manually create foreign key indexes to optimize the join queries between parent and child tables. |
The INSERT statement conflicted with the FOREIGN KEY constraint | Ensure that the values being inserted into the child table exist in the parent table. Alternatively, you can temporarily disable the constraint, insert the values, and then re-enable the constraint. |
Cannot add foreign key constraint | Verify that the referenced column in the parent table has a corresponding primary key or unique constraint. Also, ensure that data types match between the child and parent tables. |
Cannot add or update a child row: a foreign key constraint fails | Make sure that the value being updated or inserted in the child table has a corresponding value in the parent table. |
Introducing FOREIGN KEY constraint may cause cycles or multiple cascade paths | Disable the FOREIGN_KEY_CHECKS constraint, perform the data modification, and re-enable the constraint. |
Checking foreign key relationships
To verify foreign key relationships in your database, you can utilize system tables and execute specific queries that we are going to look into in this section of our article.
How to use system tables to check foreign key relationships
For a little bit of hands-on experience, let us use system tables and check foreign key relationships:
SELECT
FK.name AS FK_Name,
TP.name AS Parent_Table,
RFK.name AS Referenced_Table
FROM
sys.foreign_keys AS FK
INNER JOIN
sys.tables AS TP ON FK.parent_object_id = TP.object_id
INNER JOIN
sys.tables AS RFK ON FK.referenced_object_id = RFK.object_id
On executing the query above, you will get the names of foreign keys, parent tables, and referenced tables:
Examples of queries to check foreign key relationships
To list all foreign keys with their details:
SELECT
fk.name AS FK_Name,
tp.name AS Parent_Table,
rfk.name AS Referenced_Table,
rkc.name AS Referenced_Column
FROM
sys.foreign_keys AS fk
INNER JOIN
sys.tables AS tp ON fk.parent_object_id = tp.object_id
INNER JOIN
sys.tables AS rfk ON fk.referenced_object_id = rfk.object_id
INNER JOIN
sys.foreign_key_columns AS fkc ON fkc.constraint_object_id = fk.object_id
INNER JOIN
sys.columns AS rkc ON fkc.referenced_column_id = rkc.column_id
AND fkc.referenced_object_id = rkc.object_id
As a result, you will see the list of all foreign keys along with the respective parent tables, as well as the tables and columns they reference:
To find foreign keys in a specific table:
SELECT
fk.name AS FK_Name,
tp.name AS Parent_Table,
rfk.name AS Referenced_Table,
rkc.name AS Referenced_Column
FROM
sys.foreign_keys AS fk
INNER JOIN
sys.tables AS tp ON fk.parent_object_id = tp.object_id
INNER JOIN
sys.tables AS rfk ON fk.referenced_object_id = rfk.object_id
INNER JOIN
sys.foreign_key_columns AS fkc ON fkc.constraint_object_id = fk.object_id
INNER JOIN
sys.columns AS rkc ON fkc.referenced_column_id = rkc.column_id
AND fkc.referenced_object_id = rkc.object_id
WHERE
tp.name = 'YourTableName' -- Replace with the actual table name
The query output will contain the same set of columns as the previous one — foreign keys, parent tables, referenced tables, and their corresponding columns — yet specifically for a singular table.
To find tables that have foreign key references to a specific table:
SELECT
tp.name AS Referencing_Table,
rfk.name AS Referenced_Table,
fk.name AS FK_Name
FROM
sys.foreign_keys AS fk
INNER JOIN
sys.tables AS tp ON fk.parent_object_id = tp.object_id
INNER JOIN
sys.tables AS rfk ON fk.referenced_object_id = rfk.object_id
WHERE
rfk.name = 'YourReferencedTableName' -- Replace with the actual referenced table name
This query will help you identify which tables in your database are connected to a specific referenced table through foreign key relationships.
Conclusion
Wrapping up everything we have just covered, understanding foreign keys is fundamental to maintaining data integrity in relational databases. They establish important relationships between tables, ensuring consistency and accuracy in our data. Tools like dbForge Studio for SQL Server provide a user-friendly interface to manage foreign keys effortlessly. Download a 30-day free trial today and experience firsthand how dbForge streamlines the process of working with foreign keys, enhancing the efficiency and reliability of your database operations.